In the previous article, we saw how we can determine a Prime Number using Python logic and syntax. In this article we will explore All Divisors of a Number in Python. Imagine that we have a tower of height 12 using equal size blocks, we need to find the size of all the blocks that can make the height exactly 12. One possible way to do this would be to fill the tower with blocks of size 1, another would be to fill it with just a block of size 12. All the possible ways would include:
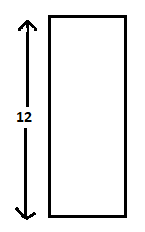
All the possible ways would include:
- Twelve blocks of size 1(12×1)
- Six blocks of size 2(6×2)
- Four blocks of size 3(4×3)
- Three blocks of size 4(3×4)
- Two blocks of size 6(2×6)
- One block of size 12(1×12)
However, if the tower was of size 13 there would only two ways(1×13) and (13×1).
Implementation of program to determine divisors in Python
We take a number n from the user, run a loop from 1 to n. If the number divides n we print it, if it does not we simply ignore it.
Using For Loop:
n=int(input("Enter n: "))
for x in range(1,n+1):
if n%x==0:
print(x)
Output:
Enter n: 12
1
2
3
4
6
12
Using while Loop:
n=int(input("Enter n: "))
x=1
while x<=n:
if n%x==0:
print(x)
x=x+1
Output:
Enter n: 12
1
2
3
4
6
12
So, we have seen the two ways in which the divisors of a number can be obtained in Python. In the next article we will look at optimized solutions for some of the programs we have already done.
2 thoughts on “All Divisors of a Number in Python”