In the previous article we saw about Fibonacci Numbers, now we will learn how we can check if a user given number is a prime number. Let us look at a program involving prime numbers, we are given a tower of height 91 which is made using some blocks. The blocks should all be of the same height.
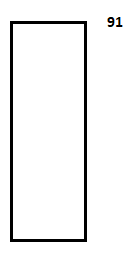
We can use the 7 blocks of height 13 to fill up the tower(7*13) using equal height blocks:
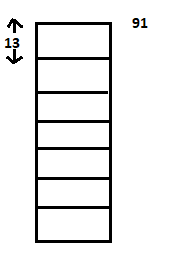
Let us now try solving our second puzzle, we have a tower of height 101 and need to fill it with equal size blocks.
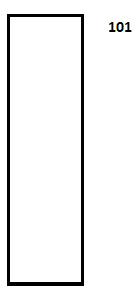
The answer to this puzzle is no, we cannot fill up the tower by using fixed equal size blocks. There is no number from one to 101(besides 1 and the number itself) that can completely divide 101. So, the number is what we call as prime number.
Implementation of Program to Determine Prime Number in Python
We need to accept the user input number, and then determine through conditionals if the number can be completely divided by any number besides 1 and itself.
n=int(input("Enter a number : "))
if(n <=1):
print("No")
else:
for i in range(2,n):
if(n%i==0):
print("No")
break
else:
print("Yes")
Output:
Enter a number : 7
Yes
The program loop checks if any number from the range to the entered number itself is completely able to divide the entered number. If no number with this particular range is found, the program prints “No” and the break statement is executed to terminate the loop. If a number is found matching within the loop the program prints “Yes” as the result.
Hence we have seen the logic with which we can determine whether a number is a prime number in Python. In the next article, we will look at All Divisors of a Number Python program.