In this tutorial post, I am going to cover all the core java concepts that is necessary for getting started in the java programming language. I will start from the bare basics assuming that this is the first time you will be interacting with java programming language. After completing this tutorial you will be ready to go in any java field or framework like competitive programming Android, back-end, etc. So, let’s get started by setting up the java coding environment in your computer.
Table of Contents
Setting up Java coding environment
For running java code in your computer, you basically need two things. First is JDK (java development kit) and second thing is an editor. JDK is something that helps your computer to understand the Java codes. For editor we will be using Eclipse. Why eclipse? because it has both editor and compiler. It is a good choice. You can also go with other softwares like Intellij Idea, STS, etc.
Installing JDK
Installing JDK is really simple. Just follow the below steps and you are good to go.
Step 1: Search on google “download JDK” or directly go to JDK download page by clicking here.
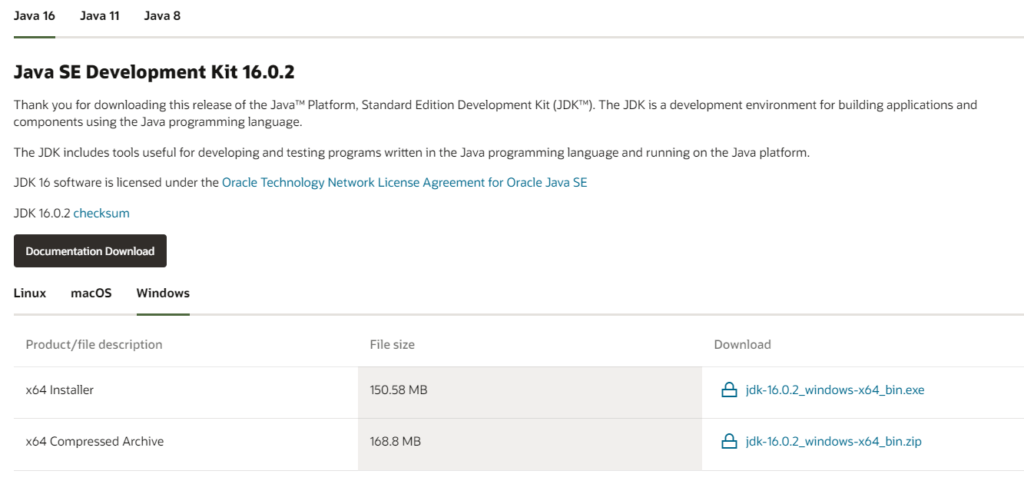
Step 2: Select a jdk version greater than 8. As a lot of things changed and adopted in java after the 8th version. So prefer going with 8+
Step 3: Download the installer or compressed archive based on your operating system
Step 4: Install it like a general software by clicking next, next.
Next thing we need is an editor, for that we chosen eclipse.
Installing and configuring eclipse for Java development
Eclipse is one of the best IDE (Integrated development environment) for Java. It comes with both editor and compiler. Moreover, it can also be used for other programming languages like C/C++.
Download the eclipse IDE by clicking here. After downloading double click to install it.
Next eclipse will ask to choose a configuration. Search for “Eclipse IDE for Java developers” and click on it. Like below image
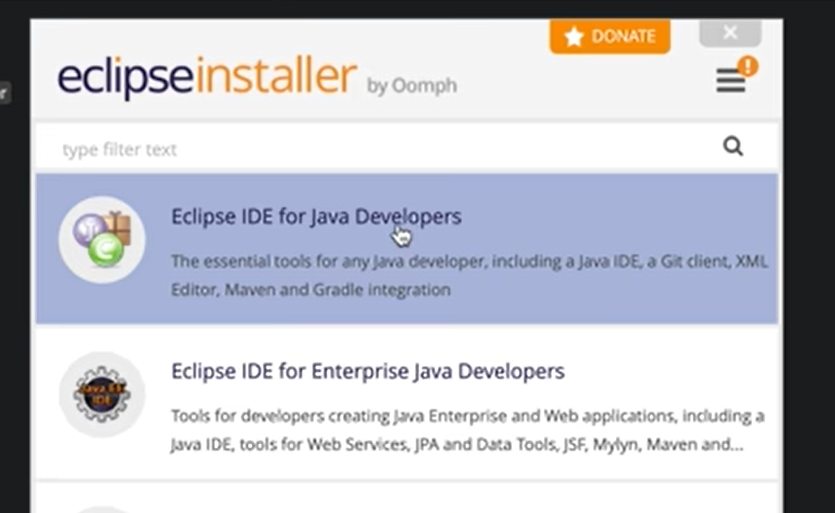
Now eclipse will ask you for JDK path, which will be basically used for compiling and running your java code. Generally it auto detect the JDK path. Then, select an installation folder and click on Install.
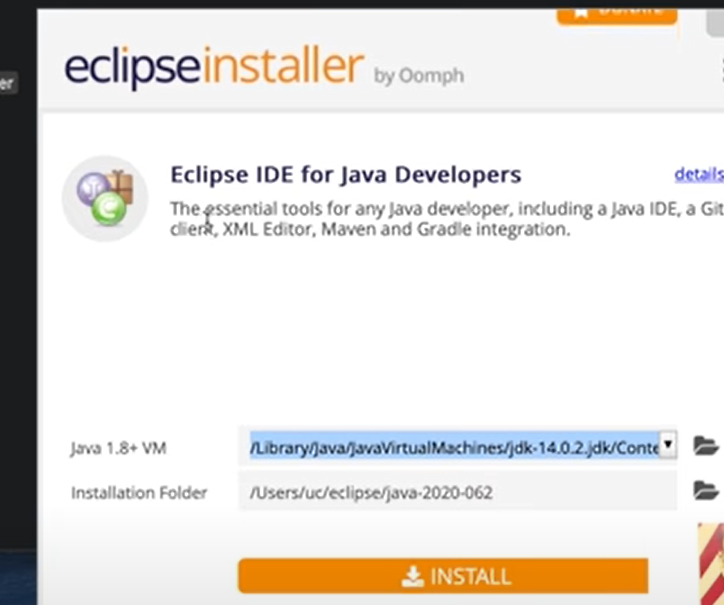
Now you are all set up with the IDE.
Java Comments
Comments are basically static texts for describing things inside a program. Comments are ignored by the compiler. Like and C++, in java also we use ‘//’ for commenting.
Class, Method and Object in Java
Java is a complete object oriented programming language (OOPS). Unlike functional programming style OOps is a different style of writing programs. At first it may sound complicated, but trust me it will make your life very easy a you get a grasp of it. Now, let’s see the basic pillars of OOPS in java that are classes, attributes, methods, and objects.
What is Java class?
Class is a complete blue print of an entity. It can be compared with the map of the house or a design of a car. Classes consists of variables(attributes), methods. Classes can be public or private. A public class can be accessed from anywhere opposite to a private class.
public class Codekyro{
int x = 5;
}
// public represents access level of the class
// class is a keyword to define classes
// CodeKyro is the name of the class
As per conversion, class name start with capital letter. A java file must have a class named as file name. A java file can only have one public class, rest other classes must not be public. A class is also called as object constructor. It is very similar to structures in functional programming.
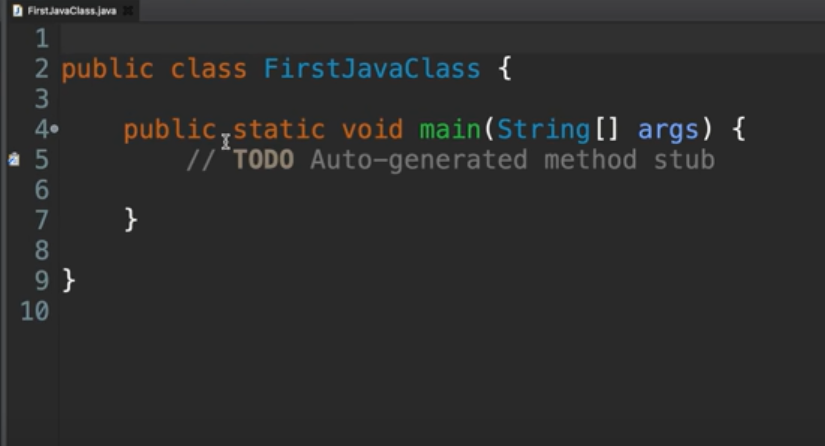
What is Java class Attribute?
Class attributes are basically variables created inside a class.
public class Main {
int x = 5;
int y = 3;
}
// Here x and y are attributes
What is Java class method?
Methods are basically functions inside a class, for doing a certain task. They are basically block of codes that only run when you call the method. For example, brake functionality in a car design. We can also pass arguments in method.
public class Codekyro{
// returnType functionName (arguments) {}
static void myMethod() {
System.out.println("Hello World!");
}
}
// void is the return type of the method
// myMethod is the name of the method
// static keyword before void can be used to call the method without making a object of this class
What is Java class Object?
Object are instance of a class. A good analogy could be, suppose a class is the map of a house then the real house build using that map is the object.
To create an object of a class, specify the class name, followed by the object name, and use the keyword new:
public class Codekyro {
int x = 5;
public static void main(String[] args) {
Codekyro myObj = new Codekyro();
System.out.println(myObj.x);
}
}
// An object named myObj of Codekyro class is created
Hello world program in Java
Now, its time to write your first program in java. Open eclipse IDE and in top left corner click on File option.
Select New->Java project. Give a name to your project.
Now inside the project folder you will have two files ‘JRE system library’ and ‘src’. ‘JRE system library’ will have lots of .jar files which java provide us to support the development. Close that folder and come to src. This folder is basically where we will write all our code.
Right click on src folder then click on new and select class.
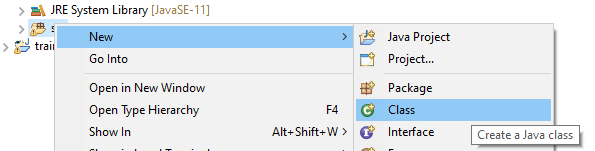
As I have already told that java is an object oriented programming language. so , we need to create a class before anything.
Give a name to your class, make sure first letter is capital. In my case I’ve given a name ‘HelloWorld’ to the class. Make sure you tick on ‘public static void main(String[] args)’. This will create a main method for you inside the class.
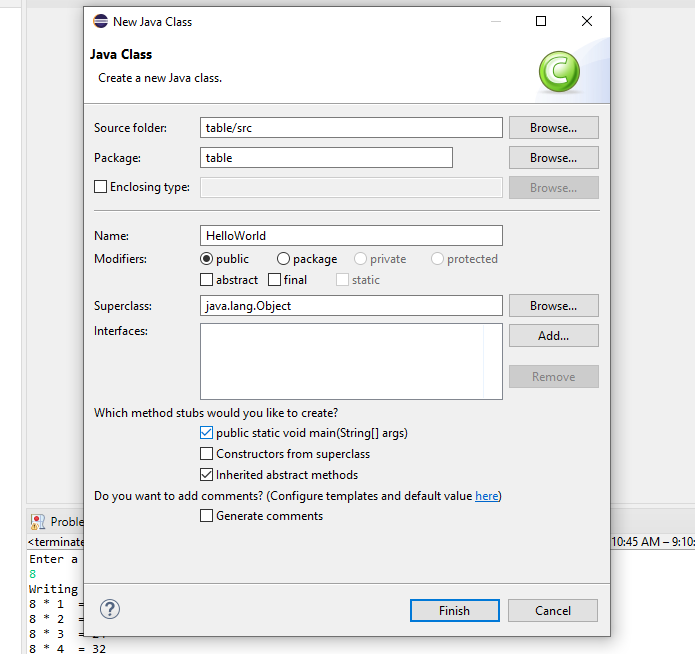
Now, use System.out.println() to print Hello world.
public class HelloWorld{
public static void main(String[] args) {
System.out.println("Hello world!");
}
}
Variables in Java
Variables are basically containers ti hold data in our program. A java variable consists of data type and a name. For naming a variable we can use alphanumeric charters and two special characters that are $(dollar) and _(underscore). A variable name is cannot start with a number. Also, you cannot use reserved keywords of java to create variable name.
public class Codekyro{
public static void main(String[] args){
int x;
x = 5;
System.out.println(x);
}
Data type in Java
For creating a variable we need to define a data type of the variable. Different data types are used to store different kind of data. Some common data types in Java are int, float, char, String, long, double, boolean, etc. Data type in Java is divided in two categories
- Primitive data type: in-build data types of java
- Non- Primitive data type: data types defined by programmer
There are 8 primitive data types are in Java int, byte, short, long, float, double, boolean, and char. A primitive type has always a value, while non-primitive types can be null .
int marks = 18;
float averageMarks = 19.345f;
char grade = 'A';
String name = "Anuj";
boolean isValid = false;
long bigNumber = 98749823749823l;
Taking Input in Java
For taking input in java we use scanner class. We have to use different methods of scanner class to take input of different data types.
Scanner sc = new Scanner(System.in);
System.out.println("Enter your name: ");
String name = sc.nextLine(); // For taking String input
int num = sc.nextInt(); // For taking integer input
float avg = sc.nextFloat() // For taking floating number as input
System.out.println(name);
System.out.println(num);
Operators in Java
Operators are for doing different type of operations in programs. their are four types of operators present in Java. Those are Arithmetic operators, Logical operators, Assignment operators, Bit-wise operators, etc. Let’s see one by one.
Arithmetic operators in Java
Arithmetic operators are doing simple mathematical operation like addition, subtraction, etc in a java program. Arithmetic operators in java are:
+: Addition
-: Subtraction
/: Division
*: Multiplication
%: Modulo (return the reminder of the division)
++: Increase by 1
--: Decrease by 1
Conditional Statements in Java (if-else)
Condition statement are basically used for running a code block if certain condition is met else run a different code block.
int age = 15;
if ( age > 18 ) {
System.out.println("you can vote");
} else {
System.out.println("you can not vote");
}
// For adding more conditions we use else if()
Output: you can not vote
Conditional Operators in Java
There are basically 6 conditional operator in Java
> : greater than
< : less than
>= : greater or equal
<= : lessor equal
== : equal
!= : not equal
Logical Operators in Java
Logical operators are mostly used to connect different conditional statements. They act same as logic gates we learnt in earlier classes. Their are three basic type of logical operators:
&& : AND(true if both the statements are correct else false
|| : OR(true if any statement is true)
! : NOT(reverse the boolean)
// Example
if (age > 55 || age < 5){
System.out.println("You are not allowed to marry go round");
else{
System.out.println("You are allowed to marry go round");
}
Switch in Java
Switch cases are basically an alternative of conditional statements. Suppose a program have a lot of conditions before the output. Then, in those cases, it is advisable to use switch cases as it make code more readable and clear. Below example will clarify how to use switch cases:
// A program using If-else
char grade = 'D';
if(grade == 'A') {
System.out.println("your grade is very good");
} else if (grade == 'B') {
System.out.println("very good, keep learning");
} else if(grade == 'D') {
System.out.println("keep improving");
} else {
System.out.println("invalid grade");
}
//Same program using switch case
char grade = 'R';
switch(grade) {
case 'A':
System.out.println("your grade is very good");
break;
case 'B':
System.out.println("very good, keep learning");
break;
case 'C':
System.out.println("Nice keep going");
break;
default:
System.out.println("wrong argument");
}
Note that after every case, their is a break statement written. This is to prevent the further execution of code if the case is matched. If it was not their java will execute all statements below it.
Loops in Java (for, while, do-while)
If you want to execute a certain block of code multiple time then loops can help you. Their are basically three types of loops exist: for, while, do-while. For and while are the most used ones.
//for, while, do-while
// for(;;)
for(int i = 0; i < 10; i++) {
System.out.println("Codekyro" + i);
}
// while(;;)
int a = 23;
while(a <= 100) {
a++;
if ( a == 65 ) continue; //Terminating conditions
System.out.println(a);
}
// do-while - code wil run first time for-sure
int a = 23;
do {
System.out.println(a);
a++;
} while(a > 100);
Array in Java
Array is basically a container that can store data of same data type. Indexing of array starts with 0. That means first value of the array is stored at zeroth position(index).
//array
// 23, 12, 56, 34, 99
int marks[] = new int[5]; // Array declaration
marks[0] = 23;
marks[1] = 12;
marks[2] = 56;
marks[3] = 34;
marks[4] = 99;
int marks[] = {23, 12, 56, 34, 99, 12, 34}; // Both declaration and assignment
// For iterating through the values of array we use for loop
for(int i = 0; i<=marks.length; i++) {
System.out.println(marks[i]);
}
Multidimensional array in Java
We can make multidimensional array in Java as per our need. Below code depicts a 2d array in java.
int a[][] = new int[2][3];
int a[][] = {
{
1, 2
}, {
3, 4
}
};
System.out.println(a[1][0]);
Exception Handling in Java
Sometime while coding we are unsure that whether the block of code will give correct output or not. In cases where we are unsure the correctness of the program and their could be chance of whole program to crash, we use try, catch. And in the catch block we put different kind of exceptions to know the reasons of crash.
Their are a lot of exceptions exist in Java.
int a[] = new int[3];
try {
System.out.println(2/0);
} catch(ArrayIndexOutOfBoundsException e) {
System.out.println("Error in program");
System.out.println(e.getLocalizedMessage());
} catch(ArithmeticException e) {
}
// To catch any type of exception
catch(Exception e) {}
1 thought on “Complete Java Tutorial for Beginners”