In the previous article we have explored Geometric Progression in Python. Now we will start looking at various common programs to further our understanding of Python such as Sum of Natural Numbers, Finding Last Digits or Days Before N number of Days. In this article we will explore the concept of Sum of Natural Numbers in Python.
How to handle Sum of Natural Numbers in Python
A person saves 1 rupee on day 1, 2 rupees on Day 2 and Three Rupees on Day 3. We need to find out how much money the person saves after 10 days.
A simple approach at solving this problem would be to find out the sum of all numbers from 1 to 10 is (1+2+3+4+5+6+7+8+9+10) which gives 55.
While this simplistic approach would work for smaller ranges, it would be cumbersome for a wider range like 1-100.
A loop which would still work, would involve more time to process a result from a wider range of numbers. There is a much more direct and straightforward way of approaching this problem through the form of a formula:
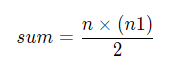
Going by the values of the formula, n=10
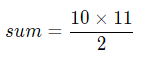
Hence , sum= 55
#Python program for Sum of n Natural Numbers using for loop
n=int(input("Enter n: "))
for i in range(n+1):
sum = sum + i
print("Sum is:" , sum)
Output:
Enter n: 10
Sum is: 55
#Python program for Sum of Natural Numbers using formula
n=int(input("Enter n:"))
sum = n * (n + 1) /2
print("Sum is:" ,sum)
Output:
Enter n:10
Sum is: 55.0
The expression for the sum variable is evaluated by the compiler as:
= 10*11/2
= 101/2
= 55.0
We get the floating point value of 55.0, Alternatively specifying double slash(//) rather than a single (/) slash would return back a rounded result.
#Python program for Sum of Natural Numbers
n=int(input("Enter n: "))
sum = n * (n +1)//2
print("Sum is:" ,sum)
Output:
Enter n: 10
Sum is: 55
In the next article we will have a look at solving and implementation for another common problem in the Python language.